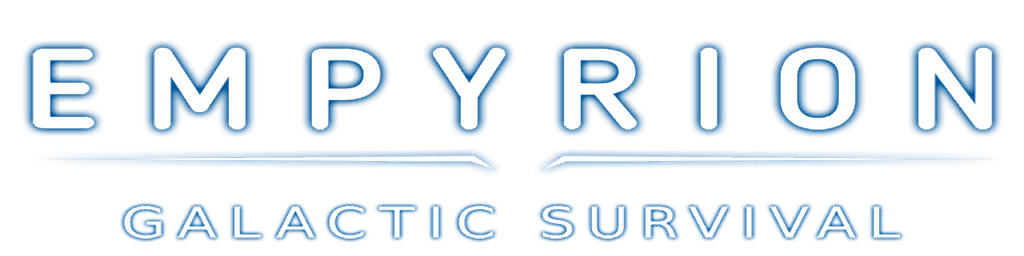
The Legacy Execute shouldn't be used anymore, so you can hide it, to save room for important stuff. However, no matter what you set here, if it is still used, it will display for compatible reasons.
Once you exported your ECF and CSV Dialogues in eWCCT (e.g. with the hotkey CTRL + S), you have to reload them ingame with the console command ds reload twice. First time for the .ecf file, second time for the .csv file.
Ingame you then set in creative mode or with godmode invisible the Dialogue state to the proper NPC Dialogue block.
If you want to test your Dialogues on a player structure use the godmode fly command.
If you get an error, most of the times it is telling you exactly what went wrong. If it doesn't, use the ds dump command. Type help ds to get more information.
Since the list below can become very long, you can cluster Dialogues visually together by having different background colors. However you have to follow a specific syntax to achieve that, so eWCCT can listen on it.
Example: Mission_BJ_Init
Hint: if you don't like the colors, just press F5 to get new ones.If set to 'False' then this item is not dropped on player death. Use for important story items that players shall not lose on death. Default is set to 'True'.
When working with conditional text variables it's very helpful to directly see what Loc string means what.
Sets the Market price of a custom Token. That can then be used in the NPCTraderConfig.ecf.
Set the name of the Dialogue. This needs to be set with godmode invisible or in a Creative game ingame by pressing F on the NPC entity.
Use NO spaces. Use camelcase or underscore or minus as separator.Set the name of the Token. It can be colorful but no breakline.
Use 'NPCName' to override or change the default entity/blockname in the dialogue header element; Needs to be set in the FIRST element of the dialogue!
With eWCCT v3.0.0 you can now export and import comments into eWCCT.
Only condition for importing comments is that they start with "#:" and are wrapped
in " ".
Example:
#: "This is a comment"
Only then eWCCT can assign it to the proper field. Sometimes I found it useful to describe a dialogue / token state in terms of meta data so I can find it later back again (where did I set the Dialogue / Token state, in what POI, what playfield, etc.)
If true, the game removes the Token once it's used. For example on a door.
How to use:
It is possible to set your own custom Icons for Tokens!
Note: ONLY .png files work!
Note: You have to relog / restart your savegame to apply the changes.
Note: You have to re-import all images in eWCCT every time you clear your Localstorage. After that they stay even if you close your browser.
The Token Id is the Lock Code ingame. It can be a number from 1 to 9999.
Use to let the NPC/Questgiver talk to the player
You can output variables in brackets like so {PlayerName}.
You can use @"type""time" to specialize your Dialogue Output even more.
"type" can be:
Example: @q0 Hello @w2 Player. @p2 @d0 Come back soon. -> This will insert a 1 second pause between Hello and Player and display "Come back soon." on another page after 1 second. "Come back soon" will be displayed instantly then. This Dialogue can't be closed by the player manually anymore.
You can show a dialog for inputting integer, float or text from players. Text strings can be up to a length of 30 characters for now. For more info check out the Dialogues-config-and-examples.txt file.
Type for the Input property. int, float or string is allowed.
What text should be shown as label for the input popup.
Positive Button text can be set here. Default (if you leave it empty) is "Ok".
Negative Button text can be set here. Default (if you leave it empty) is "Cancel".
Note: OkButton must be filled.
Use for dialogues where the user makes a choices (both work in conjunction)
Set a Barking State, where the Output text will be displayed for the NPC as soon as the player moves his cursor on it. Options and Next States will be ignored in the Barking text popup.
Here you can set an existent State, where the Dialogue should return to, if you used the Return statement
elsewhere.
Or use GotoAndReset to simulate a close and open state mechanic. That way you don't need
to inherit the variables of the next state.
Here you can decide if the given dbplayerX variable should be stored with the players entity id or steam id. In MP storing the steam id is helpful to prevent players from farming Quests (rewards) multiple time via the cb:reset command - because the player gets a new entity id everytime he does cb:reset. Default is nothing=entityid.
The difference between Executes and Execute is that Execute should be seen as obsolete. Executes does the same but each line is basically a separate Execute.
If you are familiar with C# / API Modding syntax, you can use the Executes to have access to core game
functions.
In the Example below a LCD named "MyLCD" would be considered by the Execute state and a "Hello
World!" printed on it. Example:
Execute: "ILcd lcd = LocalStructure?.GetDevice<ILcd>('MyLCD'); lcd?.SetText('Hello World!');"
Note: you can chain Execute statements by just pressing Enter (new line will be automatically exported correctly with a \n).
Note: if you want to include Dialogue variables in the Editor use the syntax '' + variable. Example: SetText('' + PlayerName);
Note: for all the Execute properties go here
This field is actually not relevant for the Dialogues or the game. It's just a helper from eWCCT to search for items you want to use in combination with AddItems in a NextIf condition for example.
Usage: search for an item, select it or press enter and then press and hold the ALT key + left mouse button to copy it to your clipboard.
Note: you have to import the Config_Example.ecf or BlocksConfig.ecf or ItemsConfig.ecf from the Configuration folder: C:\Program Files (x86)\Steam\steamapps\common\Empyrion - Galactic Survival\Content\Configuration or C:\Program Files (x86)\Steam\steamapps\common\Empyrion - Galactic Survival\Content\Scenarios\YOURSCENARIO\Content\Configuration
With some C# programming knowledge you can create here specific best case solutions for your Stories or Quests. Useful in combination with the CallLater(seconds,function) feature.
Hint: use UnityEngine.Debug.Log() to output your stuff in the ingame console.
Note: for all properties go here
Globals or constants can be used in an empty Dialogue state. Usable in ALL Dialogue states afterwards,
without the need to declare them as variable.
int LotteryNumber = 7;
An exec Function is called when Option If conditions are met.
Notice about CDATA: CDATA is an useful utility when writing native C#. There you can
use double quotes as exceptions. However make SURE to wrap your code with <CDATA> </CDATA> and
only use it in Executes!
If the Next If condition is met continue with a specific Dialogue (you can also use "End" to close the Dialogue). Limit: 30
Use for automatically advancing dialogues (based on the IF condition)(both work in conjunction)
Note: to negate an expression you have to set a ! in front. For example "!IsSignalSet('mySignal') will ask if this Signal is NOT set.
Note: param1 allows you to set Sub jump routine, so you can use Return states as value then.
Define a variable as val. For example "int dayCounter". This means a variable is declared which contains or will contain integer numbers. Limit: 30 Variables.
Options are dialogue options the player can click on / interact with. Limit: 12
Functions are an advanced programming feature, that allows you to create specific desired results.
foreach (DialogueSystem.ItemInfo itemInfo in GetItems()) {if (itemInfo.Name == 'Token') return true;}
public struct ItemInfo {public string Name; public int Id; public int Count; public int Meta;}
Helper functions:
counter = 11; CallLater(1,countdown);Function:
int counter; void countdown() { ILcd lcd = LocalStructure?.GetDevice<ILcd>('lcd'); counter = counter - 1; if (counter > 0) { CallLater(1,countdown); } lcd.SetText('' + counter); }
IContainer container = OpenDevice;
VectorInt3 blockPos = OpenDevicePos;
Hybrid Functions (Properties for the Dialogue System in the BlocksConfig.ecf):
Local variables available are:
Execute a specific val. For example AddItem('itemName',Count)
Note: you can chain Executables with a semikolon.
Note: Do not use the default Execute anymore for State executions. Use the ExecuteEditor instead.
Examples:
OpenHtmlWindow('YourTrailer', 'https://www.youtube.com/embed/xyz1234lasi73?rel=0&autoplay=1', 0.26f, 0.34f, 0.48f, 0.21f, _bShowNavButtons:false, _bgColor: new Color(1.0f,0.0f,0.0f,1.0f))"Use %SHARED_DATA% to use local content to display (root/Content/Scenarios/YOUR_Scenario/.
%SHARED_DATA%/Content/Prefabs/test.png',0.1885f,0.2125f,0.6235f,0.49f,_bShowNavButtons:false,_bBlockBG:false,_bgColor: new Color(0.05f,0.09f,0.11f,0.9f));Use %CONTENT_DIR% to use local content to display (root/Content).
OpenHtmlWindow('','%CONTENT_DIR%/test.png',0.1885f,0.2125f,0.6235f,0.49f,_bShowNavButtons:false,_bBlockBG:false,_bgColor: new Color(0.05f,0.09f,0.11f,0.9f));rel=0 will make it so the videos recommended at the end are only from that youtube channel so players won't get random videos linked at the end.
Hi Creators - RexXxuS here,
Fixed an export issue when using colons in the name of Tokens.
Here you can create and change Dialogues for your NPCs. You can create immersive Story tellers or even mini games with a bit of logic.
To configure specific Tokens for specific Doors or Container you need this Tool.
Manage your Localization.csv file for fast and easy adding/changing of your keys.
If you don't want to import any Dialogues files and start from scratch, just click on the left green + to create a new Dialogue and with that a new Dialogue journey.
If you want to import your Dialogues files, go to the
Make sure to also import your BlocksConfig.ecf and ItemsConfig.ecf for proper item suggestions and more. You can basically import your whole Scenario folder and eWCCT handels the rest
Done with all of that? Select a chapter in the left Chapter Tree. Have fun!
If you want to import your Localization.csv file, go to the
If you don't want to import any TokenConfig file and start from scratch, just click on the left green + to create a new TokenConfig and with that a new Token journey.
If you want to import your TokenConfig file, go to the